- Format of the input/output file:
- Each line of the input/output file represents one
(URL, title/info) pair and consists of
text representing the URL, a comma, and text
representing the title/info. Everything up to
the first comma (but not including the comma or
leading or trailing whitespace) is considered to
be the URL; everything after the comma (again
excluding leading and trailing whitespace) is
considered to be the title/info.
Either could contain embedded whitespace.
If there is
nothing but whitespace after the comma, the title/info
is considered to be "" (the empty string).
For example, the following file:
http://java.sun.com/index.html, Sun's Java home page
http://www.javaworld.com , JavaWorld online magazine
http://www.mystery.org ,
http://www.cise.ufl.edu/~blm/cis4930,cis4930 home page
should yield four bookmark pairs (URL and title/info
in quotes here to make it clearer what is and is not
included):
- ("http://java.sun.com/index.html",
"Sun's Java home page")
- ("http://www.javaworld.com",
"JavaWorld online magazine")
- ("http://www.mystery.org", "")
- ("http://www.cise.ufl.edu/~blm/cis4930",
"cis4930 home page")
The output file is to have the same format. (It need
not be identical if the input file contained
whitespace that was ignored, as in some lines of
the above file.)
- Name and command-line arguments:
- The program is to be started either like this:
java BookmarksGUI
or like this:
java BookmarksGUI filename
where filename is the name of the (optional)
input/output file.
- User interface (overall appearance):
- The interface presented to the user should look something
like this (your interface should incorporate
the same elements, but you may vary the appearance to
suit yourself, provided you don't vary it so much
you confuse the grader):
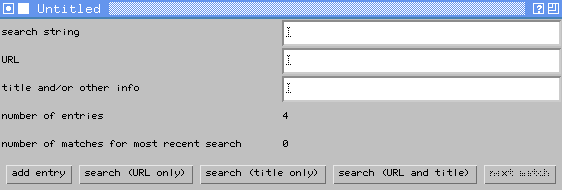
(Don't be concerned if your titlebar looks different --
the appearance of the titlebar is mostly controlled
by your system's window manager. This is what a
Java Frame looks like with the twm
window manager under X/Unix.)
Looking at the various elements in turn:
- The (fixed) text strings along the left edge
identify the three boxes for text input on the
upper right and the two numbers in the middle of
the frame.
- The three boxes are to contain user-editable text.
The user should be able to modify the contents
of these fields at any time, but no action
needs to be taken when the text is modified.
- The two numbers in the middle of the frame
represent the current number of entries
(bookmarks) in the Bookmarks object
and the
number of matches found in the most recent
search.
The former should initially be set to the
number of entries read from the input file
(if any) and should be updated whenever a
bookmark is added.
The latter should be updated whenever a
search is performed.
- The boxes across the bottom of the frame
represent buttons:
- Clicking on the leftmost button
adds an entry (bookmark), as
described below.
- Clicking on one of the middle three
buttons performs a search for
entries matching the contents of the
first ("search string") text box,
as described below.
Notice that there are three search
options -- scan URLs only, scan
titles/info only, and scan both).
- The rightmost button is enabled only
after a search and allows the user
to cycle through the results of the
search (assuming more than one match
is found), as described below.
- User interface ("add entry" operation):
- When the "add entry" button is pressed, the program
should add to the Bookmarks object an entry
(bookmark) whose URL is the contents of the second
text box and whose title/info is the contents of
the third text box. It should also update the
"number of entries" field. For example,
if "add entry" is pressed when the frame looks like
this:
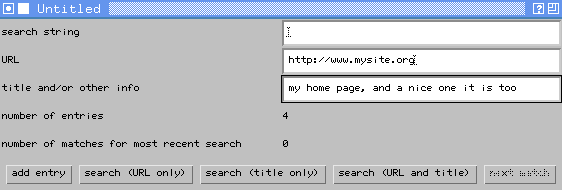
the program should add a bookmark with
URL="http://www.mysite.org" and
title/info="my home page, and nice one it is too",
and update the number of entries field, giving this:
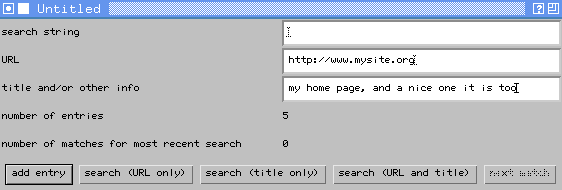
- User interface ("search" operation):
- When one of the search buttons is pressed, a search
is performed for all entries containing the
search string (contents of the first text box).
Depending on which of the buttons is pressed,
the search scans the URL parts of all bookmarks,
the title/info parts of all bookmarks, or both.
For example, suppose the Bookmarks object
contains
the bookmarks from the input-file example earlier,
plus the one added by the "add" example above,
and the user presses the "search (URL only)" button
when the frame looks like this:
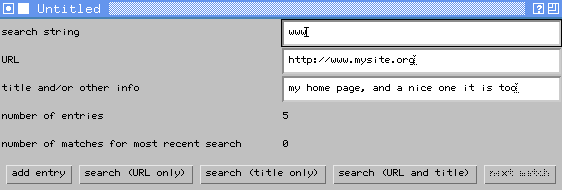
This search will find 4 matches (all entries except the
one for Sun's Java home page).
Conceptually, the result of a search is a list of L
"matches" -- bookmarks in which the search string
appears (in the URL or the title/info, depending on
the type of search). The program should display
the length of L as "number of matches for most
recent search" and then use the URL and title/info
text boxes and the "next match" button to display
the elements of L as follows:
- If L is empty, the program should leave the
"next match" button disabled and clear
(remove the text from) the
URL and title/info text boxes.
- If L is not empty, the program
should use the URL and title/info text boxes
to display an element of L. The
"next match" button should be enabled if
there are elements of L that have not yet
been displayed. Every time this button is
pressed, a new element of L should be displayed
(in the URL and title/info text boxes).
When there are no more elements to display,
the "next match" button should be disabled
again.
So, for our example search above, immediately after
the search button is pressed the frame will look
like this:
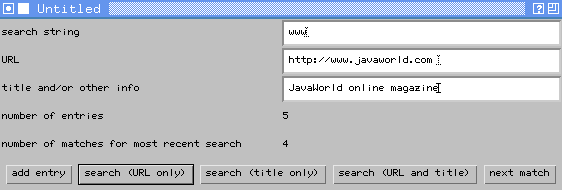
Repeatedly pressing the "next match" button will
produce this sequence of states:
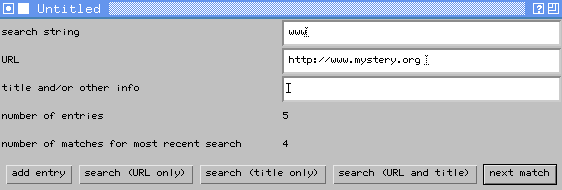
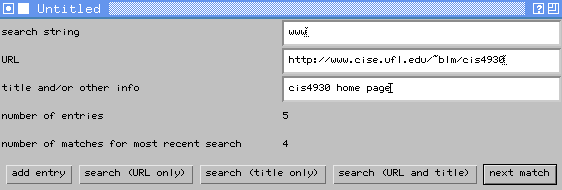
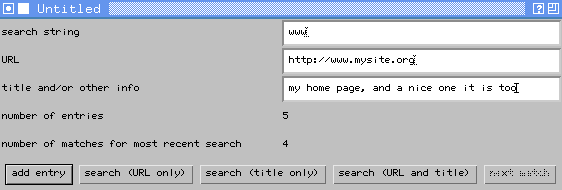
Observe that after all four matches have been
displayed, the "next match" button is disabled again.
Note that a search that finds only one match will
not enable the "next match" button.
- User interface (program termination):
- The program is to terminate (and write its output file,
if there is one) when the frame window is closed.
- Etc.:
- Note that you are not required to check the format
of the bookmarks' URL -- for this program, a URL
is just a text string. Adding some validity
checking would be an interesting extension but
is not required!